Syscoin + Node.js = Blockchain Apps!
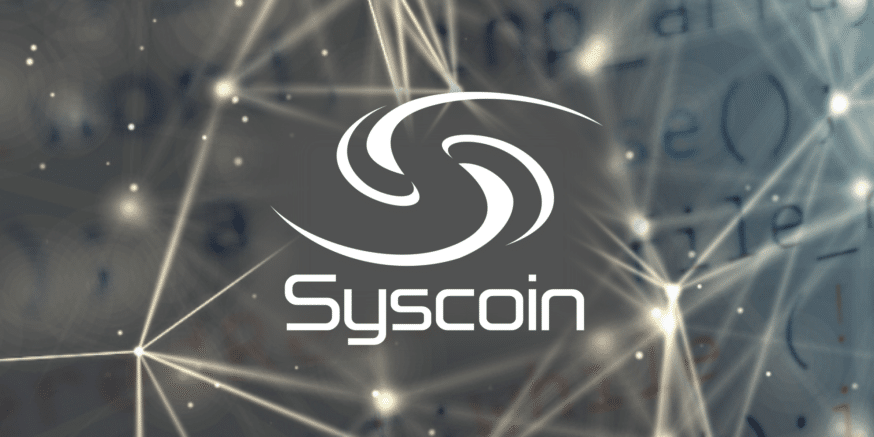
Getting Started
You will need to have syscoind
or Syscoin Core Qt running on your system and have node
/npm
installed. If you don’t have Node.js I recommend installing it via nvm
.
Syscoin Configuration
First make sure that syscoind
is configured so that you can connect to the RPC server locally. Use the example below, choosing a secret rcpuser
, rpcpassword
, and optionally changing the rpcport
. You will need to use these values later to connect to query the blockchain using Node.js later.
You don’t really need the ZMQ config for this example, but it shows how to tell Syscoin to listen on those ports should you want to implement a Node.js ZMQ client in your application :).
Before making changes be sure to stop Syscoin by running syscoin-cli stop
, update the configuration, then run syscoind
to start the process again. If you change the config file before stopping Syscoin it will prevent syscoin-cli
from being able to communicate with the RPC server properly. Changing the index values may require you to restart with syscoind -reindex
.
# server server=1 daemon=1 # indexes addressindex=1 txindex=1 litemode=0 # rpc rpcuser=u rpcpassword=p rpcport=8370 rpcallowip=127.0.0.1 # zmq listener config zmqpubaliasrecord=tcp://127.0.0.1:3030 zmqpubaliashistory=tcp://127.0.0.1:3030 zmqpubaliastxhistory=tcp://127.0.0.1:3030 zmqpubassetrecord=tcp://127.0.0.1:3030 zmqpubassetallocation=tcp://127.0.0.1:3030 zmqpubassethistory=tcp://127.0.0.1:3030 zmqpubcertrecord=tcp://127.0.0.1:3030 zmqpubcerthistory=tcp://127.0.0.1:3030 zmqpubescrowrecord=tcp://127.0.0.1:3030 zmqpubescrowbid=tcp://127.0.0.1:3030 zmqpubescrowfeedback=tcp://127.0.0.1:3030 zmqpubofferrecord=tcp://127.0.0.1:3030 zmqpubofferhistory=tcp://127.0.0.1:3030 zmqpubhashblock=tcp://127.0.0.1:3030 zmqpubhashtx=tcp://127.0.0.1:3030 zmqpubhashtxlock=tcp://127.0.0.1:3030 zmqpubrawblock=tcp://127.0.0.1:3030 zmqpubrawtx=tcp://127.0.0.1:3030 zmqpubrawtxlock=tcp://127.0.0.1:3030
Init Node App
Next create a new folder for your project and create a Node project by running npm init
and answering the questions as you see fit. The only module that is required is syscoin-core
, a cutting edge version can be found at my fork.
mkdir -p my-syscoin-app && cd my-syscoin-app npm init # answer init questions npm install -S https://github.com/doublesharp/syscoin-core.git#dev-3.0
Create index.js Script
Once syscoin-core
is installed you can use the following example to get started. Make sure that the port
, username
, and password
match the values set in your syscoin.conf
file.
const SyscoinClient = require('@syscoin/syscoin-core'); const syscoin = new SyscoinClient({ host: process.env.SYSCOIND_HOST || 'localhost', port: process.env.SYSCOIND_PORT || 8370, username: process.env.SYSCOIND_USER || 'u', password: process.env.SYSCOIND_PASS || 'p', timeout: 30000, }); async function run() { // prune expired data! const pruneStart = Date.now(); const prune = await syscoin.pruneSyscoinServices(); console.log('pruned', prune, 'in', Date.now()-pruneStart, 'ms'); // get all alias records const aliasStart = Date.now(); const list = await syscoin.listAliases(0); console.log('fetched', list.length, 'aliases in', Date.now()-aliasStart, 'ms'); // get just an array of alias names const aliases = list.map((alias) => alias._id); // ...etc process.exit(0); } run();
Wrapping Up
> node index.js pruned { services_cleaned: 0 } in 341 ms fetched 15162 aliases in 1685 ms
You can take this same basic structure and turn it into an Express app, etc, depending on the needs of your application. It’s surprisingly easy to get started building blockchain applications with Syscoin!